String resources (Android Strings) is a common format used in Android localization and translation.
Files are a regular XML files with .xml
extension.
One file contains translations for one language, including strings, plurals, and arrays.
Android Strings format is our internal successor of the Android XML file format.
Format structure
The Android Strings file format is a regular XML file with the following structure:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- This is a comment -->
<string name="hello_world">Witaj Świecie!</string>
<string name="welcome"><![CDATA[Witaj <b>%s</b>!]]></string>
<!-- This is a comment for all plurals -->
<plurals name="numberOfSongsAvailable">
<item quantity="one">Znaleziono %d piosenkę.</item>
<item quantity="few">Znaleziono %d piosenki.</item>
<item quantity="other">Znaleziono %d piosenek.</item>
</plurals>
<!-- This is a comment for all array elements -->
<string-array name="planets_array">
<item>Merkury</item>
<item>Venus</item>
<item>Ziemia</item>
<item>Mars</item>
</string-array>
</resources>
The file contains translations for one language. Each translation is defined by a <string>
tag with a name
attribute
and a localized message inside the tag. The name
attribute is a translation key that is used in the application code to
get the translation.
<string name="hello_world">Witaj Świecie!</string>
In the example above, the hello_world
key has a translation for the Polish language.
The
name
attribute is a unique identifier for the translation key, it must be unique in the file and should not contain spaces or special characters.
File structure
In Android world, translation files are usually named strings.xml
and placed in res/values-XX
directories, where XX
is a language code. For example:
res/values-en
for English,res/values-pl
for Polish,res/values-de
for German.
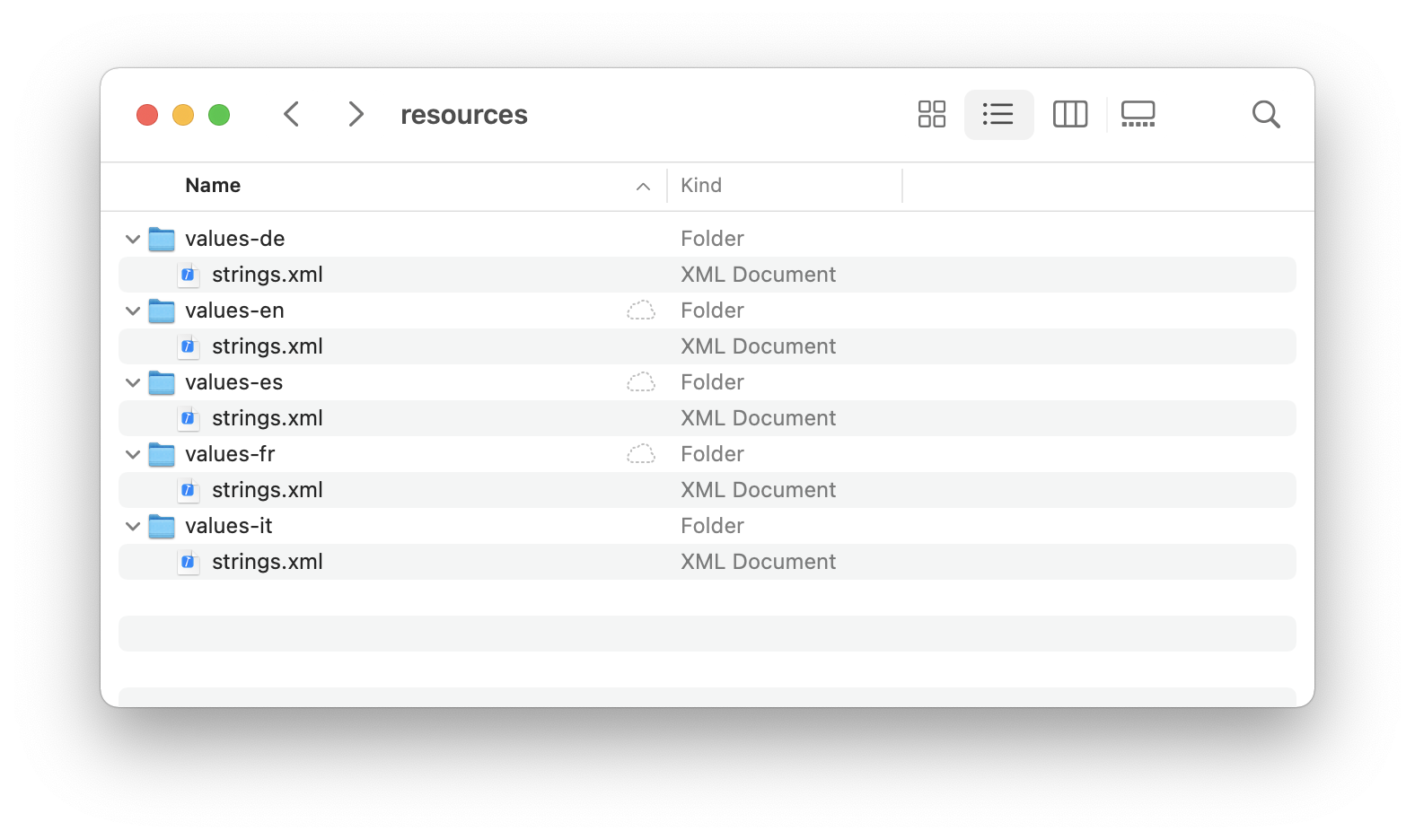
Pluralization
Pluralization is a feature that allows you to define different translations for different numbers. For example, in English
you can say "1 song" or "2 songs", but in Polish you need to say "1 piosenka" or "2 piosenki". In order to support this
feature, you need to use <plurals>
tag instead of <string>
tag. The quantity
attribute defines the number of items
that the translation is for. The following values are supported:
zero
one
two
few
many
other
<plurals name="numberOfSongsAvailable">
<item quantity="one">Znaleziono %d piosenkę.</item>
<item quantity="few">Znaleziono %d piosenki.</item>
<item quantity="other">Znaleziono %d piosenek.</item>
</plurals>
In the example above, the numberOfSongsAvailable
key has pluralized translations for Polish language.
During import SimpleLocalize converts pluralized translations to ICU message format.
{COUNT, plural, one {Znaleziono %d piosenkę.} few {Znaleziono %d piosenki.} other {Znaleziono %d piosenek.}}
COUNT
is a variable that represents the count of things,plural
is an ICU message format keyword, andone
,few
, andother
are the pluralization values.
Arrays
You can define arrays of strings using <string-array>
tag. The array can be accessed in Java code using
R.array.array_name
and in XML layout files using @array/array_name
.
<string-array name="planets_array">
<item>Merkury</item>
<item>Venus</item>
<item>Ziemia</item>
</string-array>
Translation editor shows string arrays as separate translation keys for each index.
planets_array.0
= 'Merkury',planets_array.1
= 'Venus',planets_array.2
= 'Ziemia'.
Comments
You can add comments and description to your strings, string-arrays and plurals using <--
and -->
tags. The comments
and description are not visible in the app, but they are visible in the source code. Comments above the translation keys
are automatically imported to translation editor as code descriptions.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- This is a comment -->
<string name="hello_world">Witaj Świecie!</string>
</resources>
HTML tags
You can use HTML tags in your strings. But be careful, because some tags are not supported by all Android versions. For
example, <b>
tag is supported by all versions, but <u>
tag is not supported by Android 4.0 and lower. Always use<![CDATA[...]]>
tag to wrap your HTML code.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="welcome"><![CDATA[Witaj <b>%s</b>!]]></string>
</resources>
Note:
<![CDATA[
won't show up in the translation editor, but it will be added during file export to every string that contains HTML tags.
Upload source translations
To upload source translations to SimpleLocalize, use the upload
command with the --uploadFormat android-strings
parameter, and provide the path to your strings.xml
file using the --uploadPath
parameter,
and the language key using the --languageKey
parameter.
simplelocalize upload \
--apiKey PROJECT_API_KEY \
--uploadLanguageKey en \
--uploadFormat android-strings \
--uploadPath ./values/strings-en.xml
Learn more about upload command.
Add
--overwrite
flag to update existing translations in SimpleLocalize from the imported file. Otherwise, only new translations will be added.
Export translations
To export translations from SimpleLocalize to the Android Strings file format, use the download
command with the --downloadFormat android-strings
parameter, and provide the path to the output file using the --downloadPath
parameter.
simplelocalize download \
--apiKey PROJECT_API_KEY \
--downloadFormat android-strings \
--downloadLanguageKeys pl,de \
--downloadPath ./values-{lang}/strings.xml
Please note that the --downloadLanguageKeys
parameter is optional.
If you don't provide it, all translations will be downloaded.
However, it's recommended to provide it to download only the necessary translations.
Multi-file support
If you split translations in your application into multiple files, and you want to manage them separately in SimpleLocalize. Then you can use namespaces, that will allow you to group translations into separate files, and manage them in the translation editor.
# Upload with namespaces
simplelocalize upload \
--apiKey PROJECT_API_KEY \
--uploadLanguageKey en \
--uploadFormat android-strings \
--uploadPath ./values/strings-en-{ns}.xml
# Download with namespaces
simplelocalize download \
--apiKey PROJECT_API_KEY \
--downloadFormat android-strings \
--downloadLanguageKeys pl,de \
--downloadPath ./values-{lang}/strings-{ns}.xml
{ns}
is a placeholder for the namespace name.