In this article, you will learn how to configure and use Django with SimpleLocalize to manage translations in your application. We will show you how to generate translation files, use them in your Django application, and manage PO files with the SimpleLocalize CLI.
You can also jump straight to the source code on GitHub:
Configuration
Add middleware to your Django application to handle translations.
# settings.py
MIDDLEWARE = [
...
'django.middleware.locale.LocaleMiddleware',
...
]
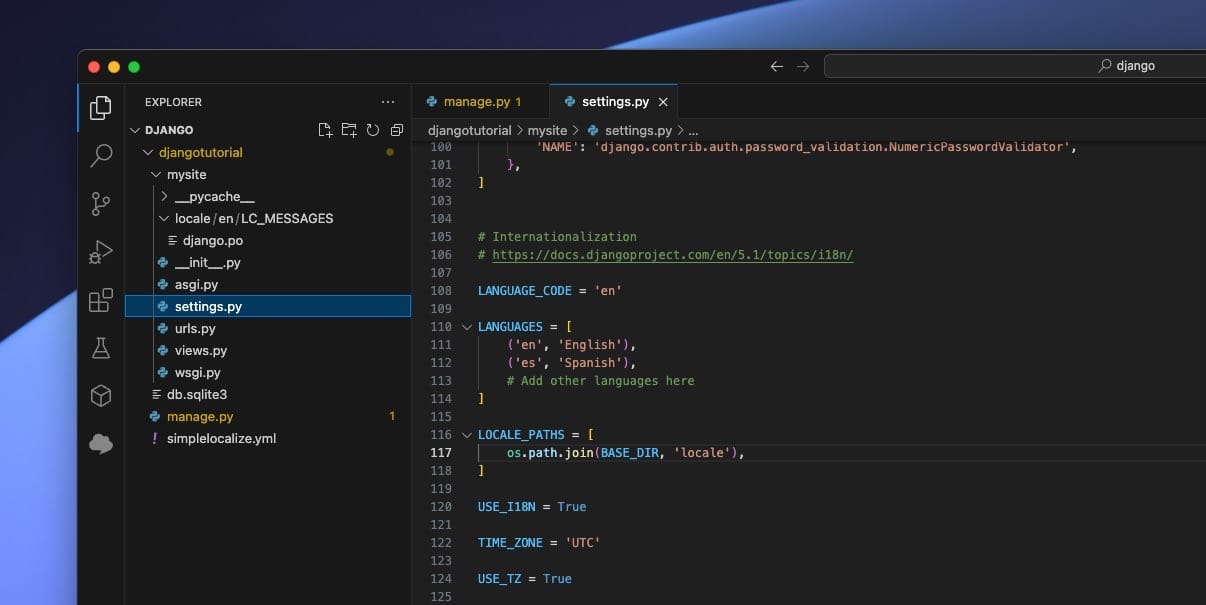
Add LOCALE_PATHS
to your Django settings to specify the location of translation files. Add LANGUAGE_CODE
and LANGUAGES
to specify the default language and available languages. Ensure that the USE_I18N
setting is set to True
.
# settings.py
LANGUAGE_CODE = 'en'
LANGUAGES = [
('en', 'English'),
('es', 'Spanish'),
# Add other languages here
]
LOCALE_PATHS = [
os.path.join(BASE_DIR, 'locale'),
]
USE_I18N = True
Generate translation files
Run the following command to generate translation files for your Django application:
$ django-admin makemessages -l en
The command will generate a locale
directory in your project root directory with the following structure:
.
├── locale
│ └── en
│ └── LC_MESSAGES
│ └── django.po
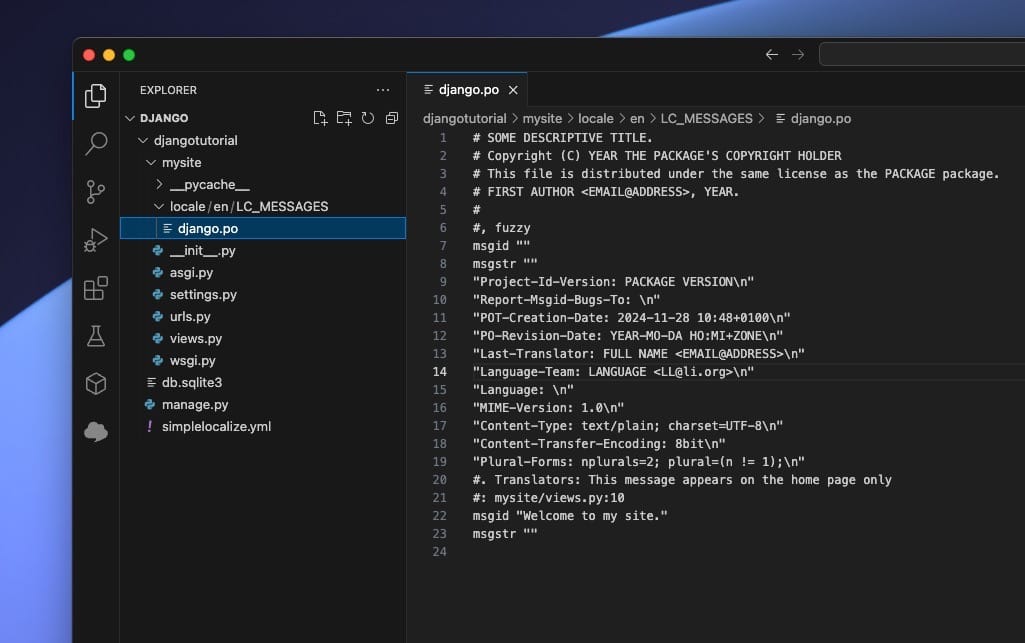
Generate MO files from PO files:
$ django-admin compilemessages
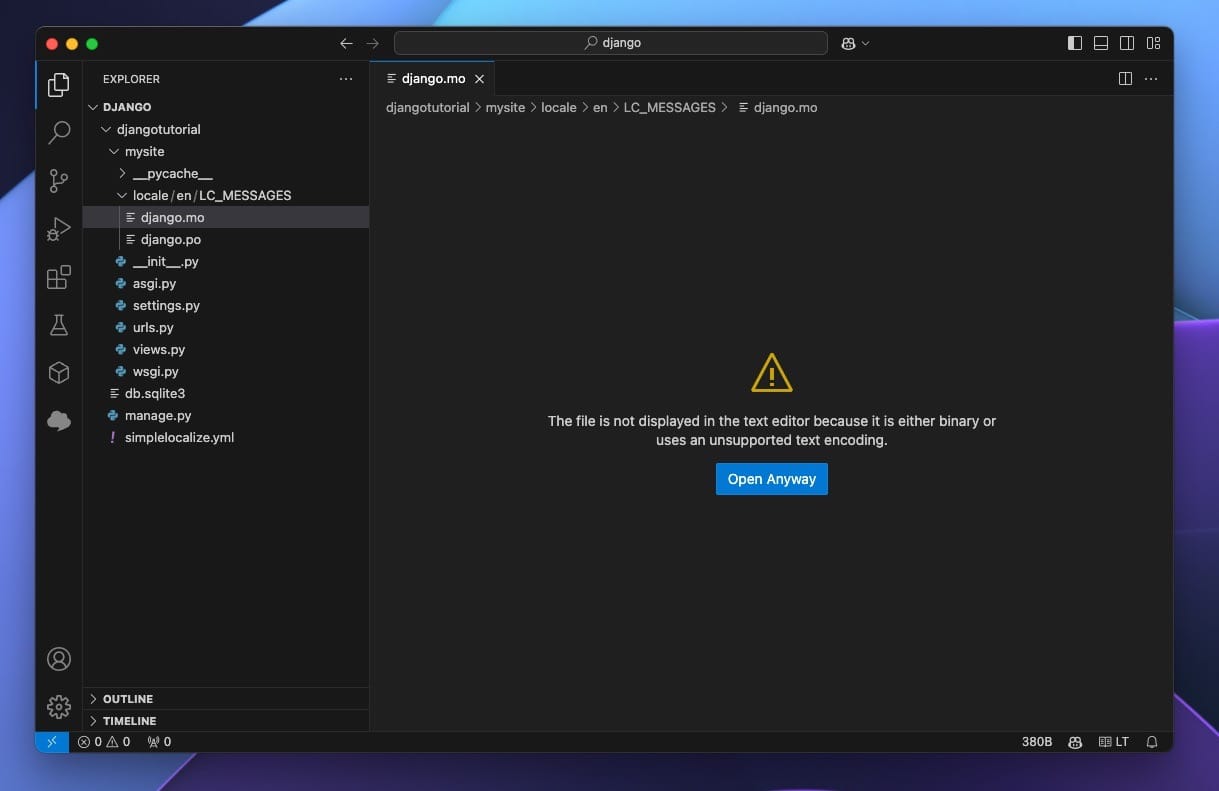
MO files are compiled versions of PO files that Django use to translate your application.
Usage
To use translations in your Django application, you can use the gettext
function.
from django.http import HttpResponse
from django.shortcuts import render
from django.utils.translation import gettext as _
from django.utils.translation import activate, get_language
def hello_world(request):
activate('es')
print(f"Current language: {get_language()}")
# Translators: This message appears on the home page only
output = _("Welcome to my site.")
print(f"Translation: {output}")
return HttpResponse(output)
gettext
function is used to get translated strings in Django. The activate
function is used to set the current language for the request. The get_language
function is used to get the current language.
We imported
gettext
as_
to make it easier to use.
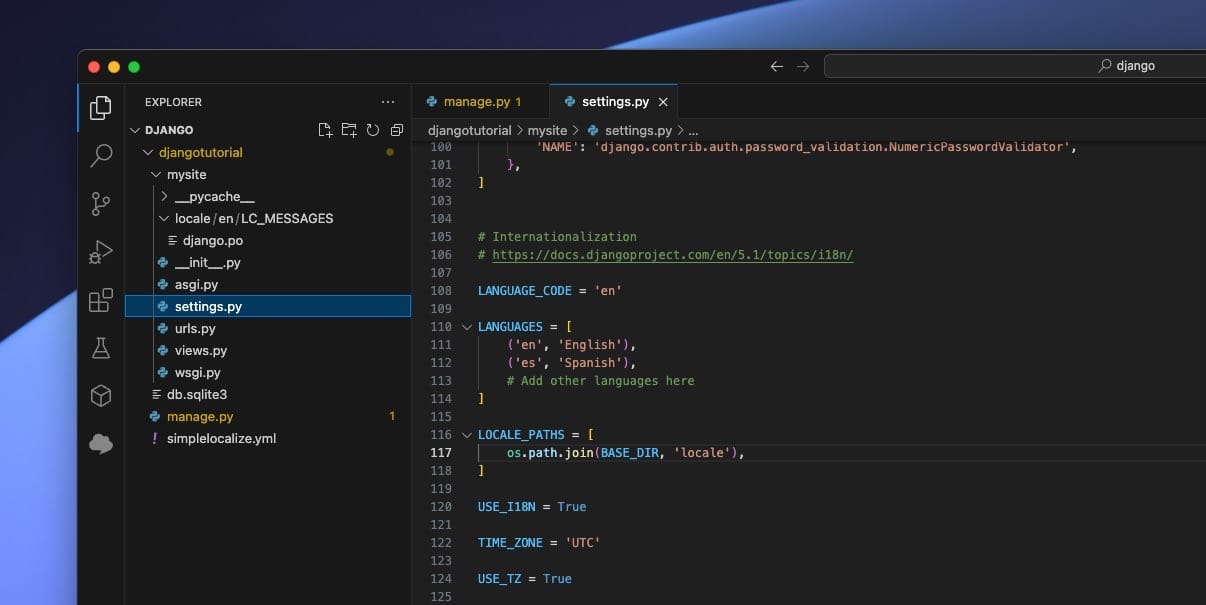
Localized response
Now, when you visit your Django /hello
, you will see the translated content based on the language you set via the activate
function,
and the gettext
function will return the translated string.
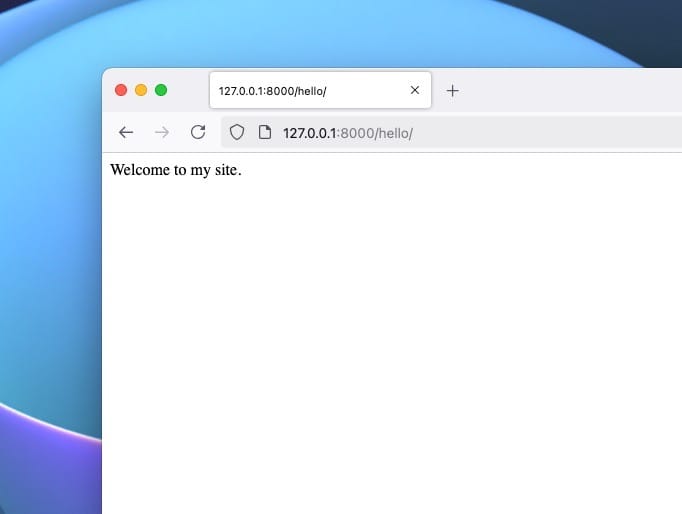
Currently, the app doesn't offer any other languages, but you can add them by generating translation files for other languages. Or use the SimpleLocalize CLI to import your source translations from PO file and export translations to other languages in the PO file format.
Managing translation files
SimpleLocalize simplifies the process of managing translation in PO files, allowing you to upload and download translations from the translation editor.
For this purpose, you can use the SimpleLocalize CLI to synchronize your Django translations between your local files and the translation editor.
Configuration
Create a simplelocalize.yml
file in your project root directory:
apiKey: YOUR_PROJECT_API_KEY
uploadFormat: po-pot
uploadLanguageKey: en
uploadPath: ./locale/en/LC_MESSAGES/django.po
uploadOptions:
- REPLACE_TRANSLATION_IF_FOUND
downloadFormat: po-pot
downloadLanguageKeys: ['pl', 'fr', 'es']
downloadPath: ./locale/{lang}/LC_MESSAGES/django.po
With this configuration, CLI will upload only English translations and download translations for Spanish, Polish, and French languages.
We use po-pot
format for both upload and download. REPLACE_TRANSLATION_IF_FOUND
option will automatically update English translations
if they are found in the translation editor during the upload process.
Upload translations
To upload translations to the translation editor, run the following command:
simplelocalize upload
After that, you can see your translations in the translation editor.
Download translations
To download translations from the translation editor, run the following command:
$ simplelocalize download
After downloading the translations, you have to compile them to MO files:
$ django-admin compilemessages
Now, you can use the translations in your Django application.